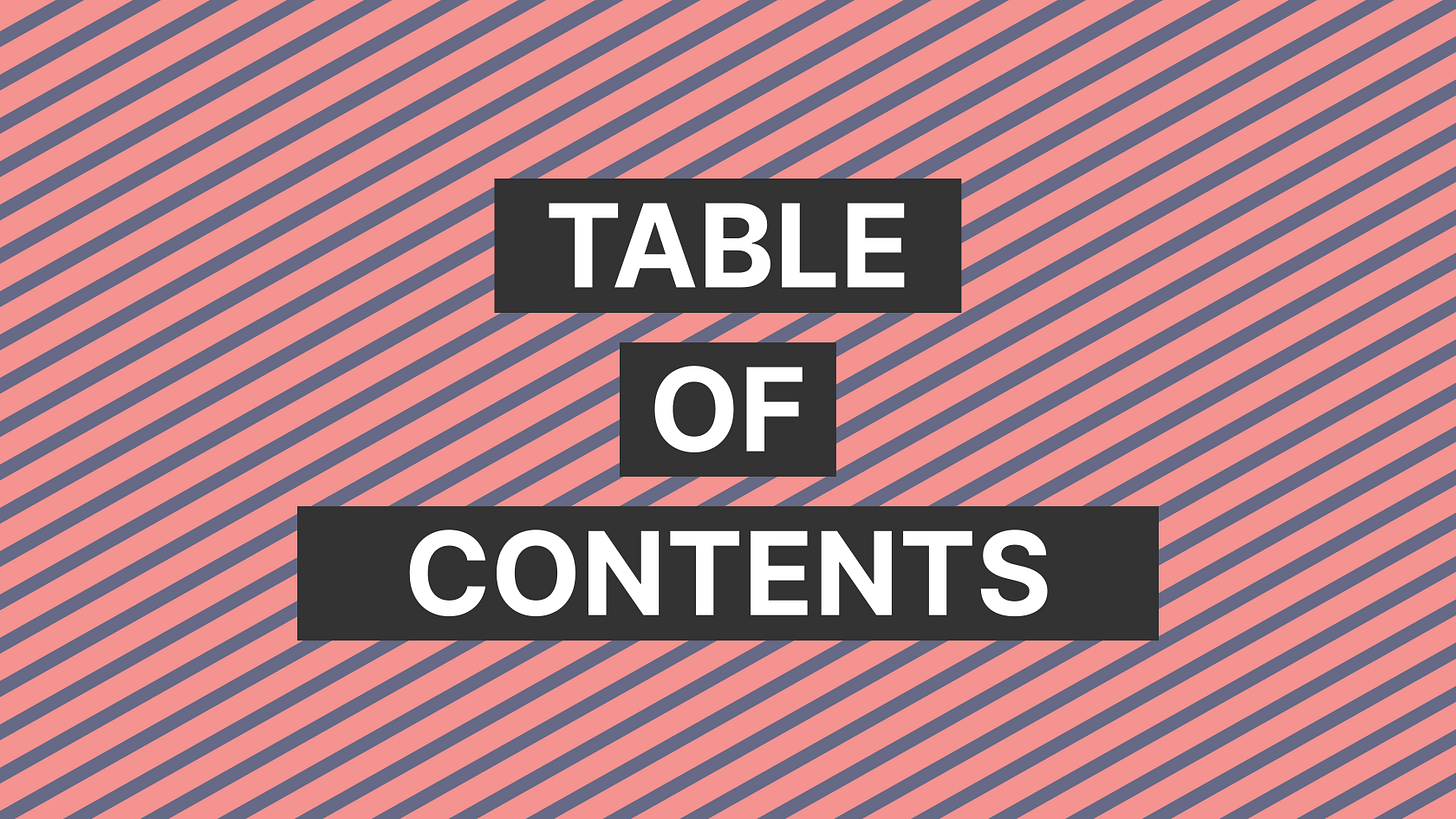
This is Python for Experienced Programmers. A weekly publication in which I provide a concise tutorial on how to use Python, aimed for experienced programmers that want to get up to speed with Python fast.
These are the topics I want to address in this publication. I won’t probably do it in order. Some of them are more advanced and I’ve been able to provide more details. If you think I’m forgetting something, or you want to suggest topics, leave a comment!
Table of Contents
Introduction (and why I’m writing this guide)
Meta Python: A quick overview of governance and community
History & important people
Python Versions
Features of the language
Python "The Language"
Python Interpreters
What does it mean to be Pythonic?
Governance and communities
PEPs: Python enhancement Proposals
Politics and Philosophy
Python usages and common libraries
Python for Web Development
Python for Data Science
Python for Scripting and Devops
Other uses
Setup and Installation
Keeping isolated Python versions withÂ
pyenv
Isolated virtual environments withÂ
virtualenv
 &Âvirtualenvwrapper
Lockfiles (and virtualenvs) with Poetry
Editors
How to actually run a Python program
Running python (and options)
Using the REPL
IPython: A better repl
Jupyter: A better better repl
Language basics
Indentation
Tabs vs Spaces (answer: use spaces) and code formatting
Comments and multiline strings (which are not comments)
Python program evaluation (hint: it's top-bottom)
Variables and names
Object identity
TheÂ
is
 operatorPython Bytecode
Data Types
Operators
Strings
Control Flow Structures
Built-in functions
File Handling
Collections
Functions
Object Oriented Programming - Part 1: The Basics
Object Oriented Programming - Part 2: Advanced
Iterators and generators
Modules and packaging
Testing in Python
Debugging and profiling
Books and recommended resources